To write about color spaces in another blog post, I needed a diagram to illustrate color spaces such as Adobe RGB and sRGB. I need a diagram showing the range each color space covers on the spectrum, with the CIE 1931 color spectrum as a background.
Given the potential inaccuracies of creating it by hand, I wondered, could I leverage ChatGPT to draw it?
This article is to share that trial and error and the results.
Can ChatGPT draw diagrams directly?
I first tested ChatGPT’s ability to draw diagrams by typing the following prompt into ChatGPT.
Create a chart showing the range of the sRGB gamut within the visible light (colors) that humans can see.
ChatGPT responded as follows.
I cannot create charts or images directly, but can provide descriptions and help you paint an image.
The visible light spectrum ranges in wavelength from about 380 nm to 780 nm and includes colors perceived by the human eye. sRGB color gamut covers a certain range within the visible light spectrum and is designed to be reproduced by most standard displays and devices.
To chart the sRGB gamut, we use the CIE 1931 color space diagram (xy color space), a two-dimensional graph with an x-axis and a y-axis that covers all colors in the visible light spectrum. In this diagram, the horseshoe-shaped area represents all colors that humans can perceive.
The sRGB gamut is represented by a triangle within this horseshoe-shaped area. the vertices of the sRGB triangle indicate the coordinates of the basic colors red, green, and blue, respectively, as follows
Red: x = 0.6400, y = 0.3300
Green: x = 0.3000, y = 0.6000
Blue: x = 0.1500, y = 0.0600
Plotting these coordinates on a CIE 1931 color space diagram and drawing a triangle allows us to visualize the sRGB gamut. The colors inside this triangle are those that can be represented in the sRGB gamut.
ChatGPT does not have the capability to draw or generate diagrams. However, image-generating AIs such as DALL-E and StableDiffusion would not be useful for this situation.
Generate the SVG
ChatGPT can generate code. It should be possible to generate any data representing a diagram in code. First, let’s try SVG. The prompt is as follows.
I see that it is not possible to create diagrams directly. So can you generate SVG or something else instead?
Then, as expected, we were able to generate SVG code.
I cannot generate SVG files directly, but I can show you how to describe SVG shapes to represent the sRGB gamut. This will allow you to create your own SVG files.
The sample code below is an example SVG that renders the sRGB gamut. This can be inserted within an SVG file or an HTML file.
<svg width="500" height="500" xmlns="http://www.w3.org/2000/svg">
<rect width="100%" height="100%" fill="white" />
<polygon points="320,165 150,300 60,60" stroke="black" fill="none" stroke-width="2" />
<circle cx="320" cy="165" r="4" stroke="red" fill="red" />
<circle cx="150" cy="300" r="4" stroke="green" fill="green" />
<circle cx="60" cy="60" r="4" stroke="blue" fill="blue" />
<text x="320" y="165" font-family="Arial" font-size="14" fill="black" dy="1.5em" dx="-1em">Red</text>
<text x="150" y="300" font-family="Arial" font-size="14" fill="black" dy="1.5em" dx="-1em">Green</text>
<text x="60" y="60" font-family="Arial" font-size="14" fill="black" dy="1.5em" dx="-1em">Blue</text>
</svg>
This code creates a 500x500 pixel SVG canvas and draws a rectangle with a white background. It then draws polygons representing the sRGB color gamut and adds circles representing the basic colors red, green, and blue. Finally, labels are drawn for each basic color.
This code uses a different coordinate system than the actual CIE 1931 color space diagram. To create an SVG using actual coordinates, you would need to apply a coordinate transformation and use the appropriate scale. However, this example shows a basic approach to visualizing the sRGB gamut.
Insert the following two lines at the beginning of the generated code, save the file as sample.svg
or a similar name, and open it in a viewer to visualize the figure.
<?xml version="1.0" standalone="no"?>
<!DOCTYPE svg PUBLIC "-//W3C//DTD SVG 1.1//EN"
"http://www.w3.org/Graphics/SVG/1.1/DTD/svg11.dtd">
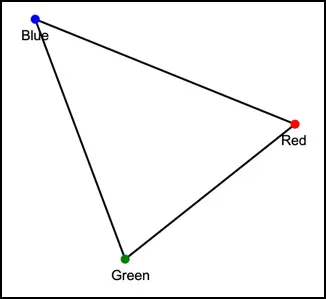
By generating code, it becomes possible to employ ChatGPT in diagram creation.
Generate the Python code
For more flexibility and control, it would be better to generate Python code and modify it by hand rather than generate SVG.
Create a code to draw a CIE 1931 color spectrum
I created a code that draws a CIE 1931 color spectrum; I entered the following prompt into ChatGPT.
Please create a python code that paints the CIE 1931 color space.
Please also color each coordinate.
The following response was then displayed.
The following is a Python program that draws a CIE 1931 color space diagram (xy chromaticity diagram) and also colors each coordinate. color-science package and matplotlib are used. Please install these libraries first.
pip install colour-science matplotlib
Next, execute the following code
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.patches import Polygon
import colour
def draw_cie1931_chromaticity_diagram():
# Draw horseshoe shape with spectral colors
wavelengths = np.arange(380, 781, 5)
xy_points = []
for wl in wavelengths:
xyz = colour.wavelength_to_XYZ(wl)
x, y = colour.XYZ_to_xy(xyz)
xy_points.append([x, y])
# Create the meshgrid
x_points = np.linspace(0, 0.8, 800)
y_points = np.linspace(0, 0.9, 900)
xx, yy = np.meshgrid(x_points, y_points)
# Create the RGB image
img = np.zeros((900, 800, 3))
for i, y_val in enumerate(y_points):
for j, x_val in enumerate(x_points):
x, y = x_val, y_val
z = 1.0 - x - y
if x < 0 or y < 0 or z < 0:
continue
xyz = np.array([x, y, z]) * 100.0
try:
rgb = colour.XYZ_to_sRGB(xyz)
if (rgb >= 0).all() and (rgb <= 1).all():
img[i, j] = rgb
except:
pass
# Plot the image
plt.imshow(img, extent=(0, 0.8, 0, 0.9), origin='lower')
# Draw the outline of the horseshoe shape
xy_points.append(xy_points[0])
x, y = zip(*xy_points)
plt.plot(x, y, color='black')
# Set up the plot
plt.xlim(0, 0.8)
plt.ylim(0, 0.9)
plt.xlabel("x")
plt.ylabel("y")
plt.title("CIE 1931 Chromaticity Diagram")
plt.gca().set_aspect('equal', adjustable='box')
plt.show()
draw_cie1931_chromaticity_diagram()
However, this code presented numerous issues. First of all, it is heavy. Also, the displayed image is all black and does not behave as requested.
So we entered the following prompt.
This code was extremely burdensome to run and did not add color, and the lines that were supposed to be written disappeared.
Then a new code was generated, but still, it was heavy, so I typed in “heavy” again, as above. Ultimately, ChatGPT generated the following code.
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.patches import Polygon
import colour
from colour.plotting import * # Required for 'plot_chromaticity_diagram_CIE1931' function
colour.plotting.plot_chromaticity_diagram_CIE1931(standalone=True, title='CIE 1931 Chromaticity Diagram', spectral_locus_colours=True)
It’s much simpler now. However, there is still a problem: plot_chromaticity_diagram_CIE1931
seems to be an old API. So I gave ChatGPT more instructions on how to fix it and finally got the following code.
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.patches import Polygon
import colour
from colour.plotting import plot_chromaticity_diagram
# Plot the CIE 1931 chromaticity diagram
plot_chromaticity_diagram(illuminant='D65', observer='CIE 1931 2 Degree Standard Observer', diagram_opacity=0, diagram_clipping_path=None, standalone=False)
Modify the generated code
The code generated using ChatGPT still has problems; repeated attempts to enter error messages into ChatGPT and have it fix them have yet to solve the problem. So it turns out that a human should review the generated code.
from colour.plotting import plot_chromaticity_diagram
This line of code is halfway through. Add the .diagramss
as follows.
from colour.plotting.diagrams import plot_chromaticity_diagram
Also, the argument of the plot_chromaticity_diagram
function is sufficient this time with standalone=True
. The modified code is as follows.
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.patches import Polygon
import colour
from colour.plotting.diagrams import plot_chromaticity_diagram
# Plot the CIE 1931 chromaticity diagram
plot_chromaticity_diagram(standalone=True)
When this code is executed, the figure appears as follows.
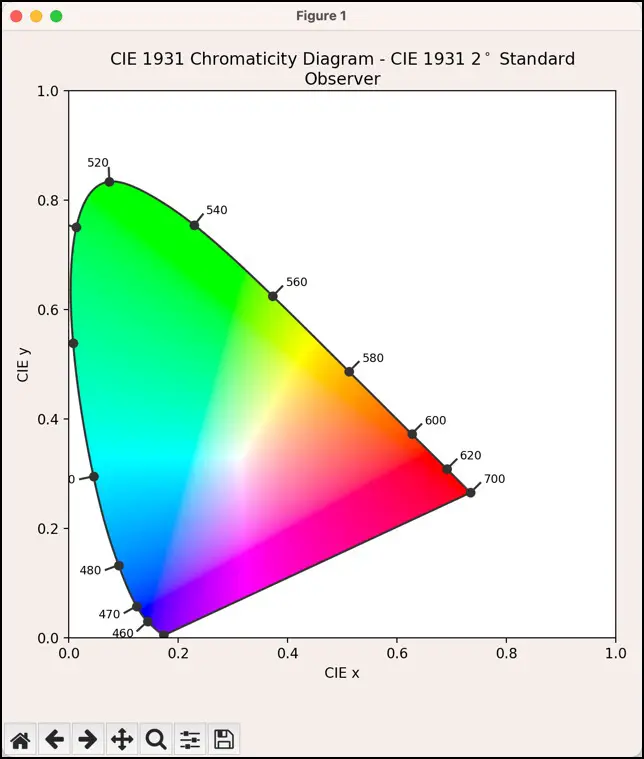
Generate code to render sRGB gamut
Next, we generate code that uses this as a background and adds code to draw the sRGB gamut. Enter the following at the prompt.
Use the following code to draw a color spectrum, and then create a Python code that triangulates the sRGB gamut on top of it.
```
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.patches import Polygon
import colour
from colour.plotting.diagrams import plot_chromaticity_diagram
# Plot the CIE 1931 chromaticity diagram
plot_chromaticity_diagram(standalone=True)
```
The following code is generated.
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.patches import Polygon
import colour
from colour.plotting.diagrams import plot_chromaticity_diagram
# Plot the CIE 1931 chromaticity diagram
plot_chromaticity_diagram(standalone=False)
# Define sRGB primaries and white point
sRGB_primaries = np.array([
[0.6400, 0.3300],
[0.3000, 0.6000],
[0.1500, 0.0600]
])
D65_white_point = np.array([0.3127, 0.3290])
# Create a polygon with the sRGB primaries
sRGB_triangle = Polygon(sRGB_primaries, edgecolor='black', facecolor='none', linewidth=2)
# Get the current axis and add the sRGB triangle
ax = plt.gca()
ax.add_patch(sRGB_triangle)
# Plot the D65 white point
ax.plot(D65_white_point[0], D65_white_point[1], 'o', color='white', markersize=10, markeredgecolor='black')
# Display the plot
plt.show()
This code works correctly. When executed, it adds a triangle indicating the sRGB gamut is as follows.
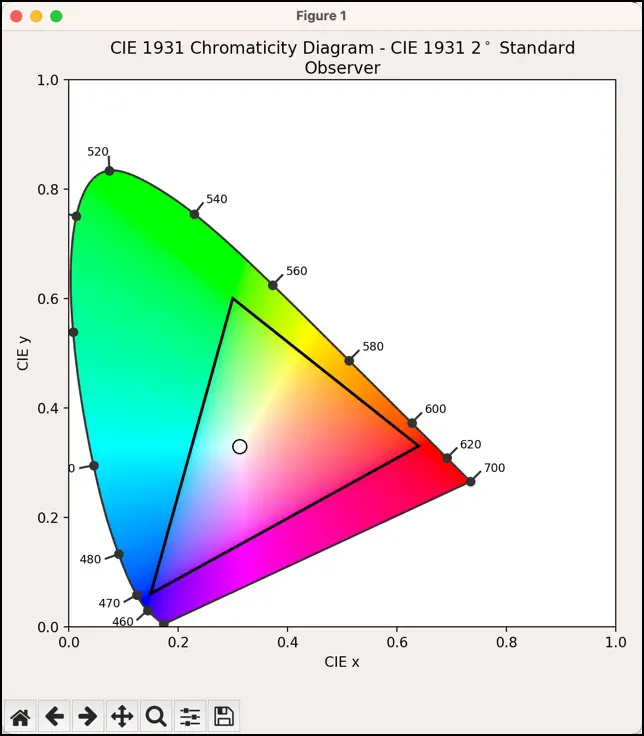
Add Adobe RGB, Display P3, and HDR10 rendering
The diagram we want to create compares several color gamuts, not just sRGB. Therefore, adding the following color space gamut rendering process is also necessary.
- Adobe RGB
- Display P3
- HDR10
So, enter the following at the prompt.
This code is a Python code that draws a diagram showing the CIE color spectrum and sRGB gamut.
Please modify this code to draw not only sRGB but also AdobeRGB, Display P3, and HDR10 gamuts based on this code.
In response, the following code is generated.
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.patches import Polygon
import colour
from colour.plotting.diagrams import plot_chromaticity_diagram
# Plot the CIE 1931 chromaticity diagram
plot_chromaticity_diagram(standalone=False)
# Define color space primaries and white point
sRGB_primaries = np.array([
[0.6400, 0.3300],
[0.3000, 0.6000],
[0.1500, 0.0600]
])
AdobeRGB_primaries = np.array([
[0.6400, 0.3300],
[0.2100, 0.7100],
[0.1500, 0.0600]
])
Display_P3_primaries = np.array([
[0.6800, 0.3200],
[0.2650, 0.6900],
[0.1500, 0.0600]
])
HDR10_primaries = np.array([
[0.7080, 0.2920],
[0.1700, 0.7970],
[0.1310, 0.0460]
])
D65_white_point = np.array([0.3127, 0.3290])
# Create polygons with the color space primaries
sRGB_triangle = Polygon(sRGB_primaries, edgecolor='black', facecolor='none', linewidth=2, label='sRGB')
AdobeRGB_triangle = Polygon(AdobeRGB_primaries, edgecolor='red', facecolor='none', linewidth=2, label='AdobeRGB')
Display_P3_triangle = Polygon(Display_P3_primaries, edgecolor='blue', facecolor='none', linewidth=2, label='Display P3')
HDR10_triangle = Polygon(HDR10_primaries, edgecolor='green', facecolor='none', linewidth=2, label='HDR10')
# Get the current axis and add the color space triangles
ax = plt.gca()
ax.add_patch(sRGB_triangle)
ax.add_patch(AdobeRGB_triangle)
ax.add_patch(Display_P3_triangle)
ax.add_patch(HDR10_triangle)
# Plot the D65 white point
ax.plot(D65_white_point[0], D65_white_point[1], 'o', color='white', markersize=10, markeredgecolor='black')
# Add legend
plt.legend(loc='upper right')
# Display the plot
plt.show()
When you run this code, you will see the following diagram.
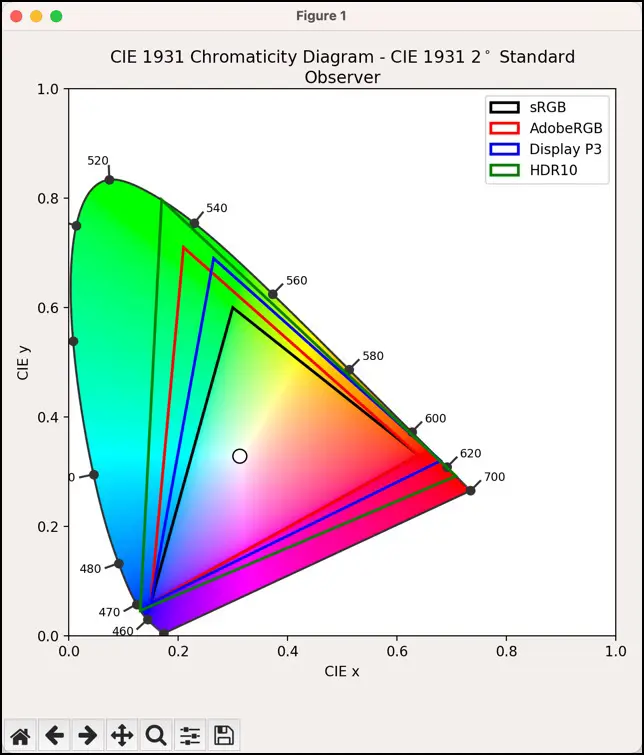
The code to draw the desired diagram is now complete.
Conclusion
ChatGPT cannot generate diagrams directly, but it can generate code to generate diagrams. By using this, you can create your intended diagram using ChatGPT.
However, the generated code may not be executable as is. Therefore, a person should verify and confirm that the generated code is OK. In other words, code review is also necessary for generated code.
Through our experimentation, we found that, at times, the code required optimization for performance. Sometimes the same prompt would not always produce the same code but different code, even the API and constants referenced.
Generative AI should be used with those characteristics in mind.